How To Create A REST Message
There is a time in every ServiceNow admins life when they want to start writing code and begin creating integrations with other systems. Maybe you need to integrate with Jira, Salesforce or another internal system at your company.
While integrations can at first appear intimidating, they are actually quite straightforward and easy to get started with.
Below I’ll cover how a ServiceNow developer would write code to make a GET REST API call, from ServiceNow.
We’ll use an example endpoint, and I’ll cover both how to use the RESTMessageV2 class using ServiceNow’s built in REST Message functionality, and without using the built in REST Management records (just using plain code).
I will cover how to consume the web services of another endpoint, and bring that data back into ServiceNow.
From here, you’d likely take the data from the JSON object, create a table and just insert the records into this table. It’s common to do this on a recurring/nightly basis, so you’re always pulling in fresh data.
ServiceNow’s class of REST Message is currently the RESTMessageV2 API, at the time of this writing. This is the class we’ll use in the examples below to make our API calls. This is an extremely extensible and simple to use class.
Covering How To Use The RESTMessageV2 API
Like most things in ServiceNow, there are multiple ways of doing the same thing.
To create a REST message in ServiceNow, you can either:
a) Create a REST Message record (preferred), and build off of this
b) Go right to scripting, don’t create a REST message record.
To send a REST message in ServiceNow, you will need to use the RESTMessageV2 API. This API allows you to send HTTP request messages and receive HTTP response messages from RESTful web services. Here is an example of how you can use the RESTMessageV2 API to send a REST message in ServiceNow:
- Create a new REST message record by navigating to REST Messages > New.
- Enter a name and optional description for the REST message.
- Under the Resource tab, enter the URL for the web service you want to call.
- Under the HTTP Method tab, select the appropriate HTTP method for the request (e.g., GET, POST, PUT, DELETE).
- Under the Headers tab, enter any HTTP headers that need to be included in the request.
- Under the Body tab, enter the request payload (if applicable).
- Click the Send button to send the REST message.
You can use the RESTMessageV2 API to send a variety of different types of REST messages, depending on your needs. You can also use the API to customize the request and response messages as needed.
So for this example, create a new REST Message record, called “Bored Activity” and click save. It will auto generate the below HTTP Method record, to GET data from the server.
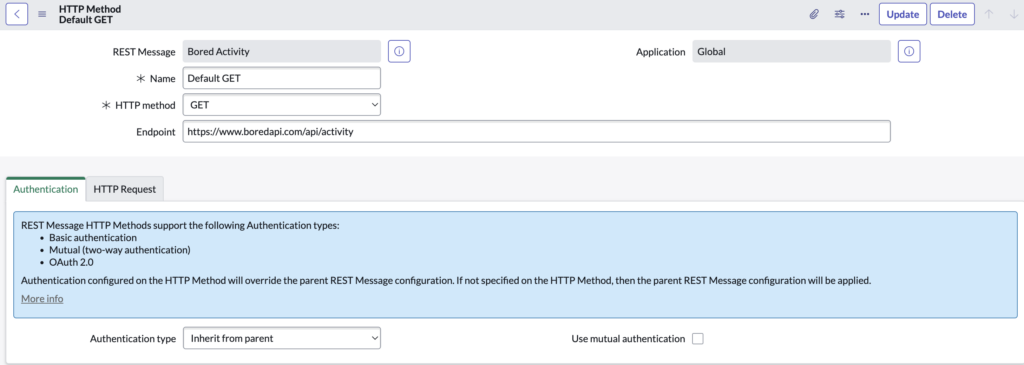
Below is the code that you can use to test the newly created REST message, using the GET method.
try {
var r = new sn_ws.RESTMessageV2('Bored Activity', 'Default GET');
//override authentication profile
//authentication type ='basic'/ 'oauth2'
//r.setAuthenticationProfile(authentication type, profile name);
//set a MID server name if one wants to run the message on MID
//r.setMIDServer('MY_MID_SERVER');
//if the message is configured to communicate through ECC queue, either
//by setting a MID server or calling executeAsync, one needs to set skip_sensor
//to true. Otherwise, one may get an intermittent error that the response body is null
//r.setEccParameter('skip_sensor', true);
var response = r.execute();
var responseBody = response.getBody();
var httpStatus = response.getStatusCode();
gs.print (httpStatus);
gs.print(responseBody);
}
catch(ex) {
var message = ex.message;
}
When the above code is executed, you’ll get a response from the server, shown below.
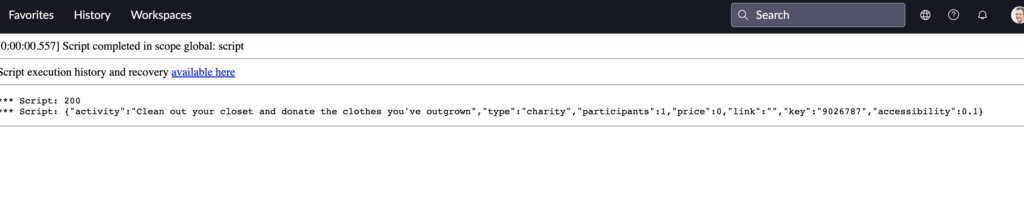
You can cut/paste the code above as an example, but you’ll have to make sure that the below line, is calling the correct REST Message record.
var r = new sn_ws.RESTMessageV2('Bored Activity', 'Default GET');
Here, we define the name of the REST Message record, and then the name of the HTTP method we’re using.
Example Of A REST Message – No Credentials
You can enter the below into any ServiceNow environment, in a background script, and you will see the response from the endpoint. www.boredapi.com is an example endpoint that you can use for testing, it has nothing to do with ServiceNow. It just serves as an example, to show if the API call is working, or not.
You’ll notice below that this example does not use any form of authentication.
// Create a new REST message
var restMessage = new sn_ws.RESTMessageV2();
// Set the HTTP method to GET
restMessage.setHttpMethod("GET");
// Set the URL for the web service
restMessage.setEndpoint("https://www.boredapi.com/api/activity");
// Send the REST message
var response = restMessage.execute();
gs.print("Status code: " + response.getStatusCode());
// Check the response status code
if (response.getStatusCode() == 200) {
// Success! Do something with the response
var responseBody = response.getBody();
var responseData = JSON.parse(responseBody);
for (var i in responseData) {
gs.print(responseData[i]);
}
// ...
} else {
// Handle the error
var errorMessage = "Error: " + response.getStatusCode() + " " + response.getStatusText();
// ...
}
This code example sends a GET request to the specified web service and checks the status code of the response. If the request is successful (status code 200), it parses the response body as JSON and does something with the data. If the request is not successful, it handles the error.
When you execute the above script, you’ll get something like this:
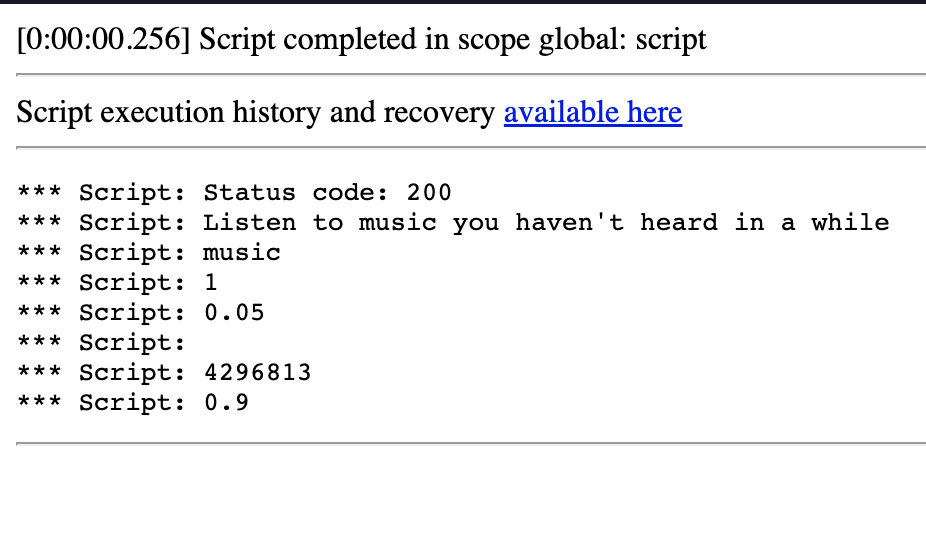
You now know how to make an API call, directly from ServiceNow.
Once you have the data in ServiceNow, you can do anything with it. Like loop through it to create records, send emails, etc.
This wraps up our coverage of ServiceNow’s REST API.
If you would like to know more about ServiceNow’s API, just drop us a message below.